First changelog
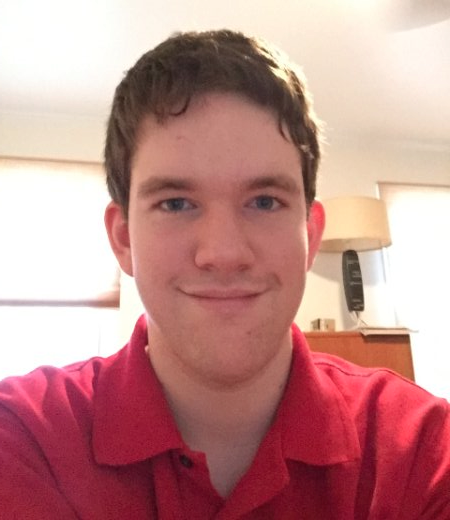
Ian here! This will be my first log of additions and changes for the game Grab It and Go. So here’s how this will work: each Sunday, I’m going to post a list of all the changes and additions I made, with a few details on what I’ve accomplished on my programming work. Occasionally, I might also have some insight to share on my own development process in making the programming for this game possible. But for the most part, this will just be a regular report on the programming and design aspects of the game. The others on the team will be tackling the graphics side of things, so I won’t be covering any of that.
So without further ado, here’s the list of new additions:
+Implemented the remote-controlled vehicle player controls:
->Acceleration/Deceleration occurs gradually - no hard stops
->Inputting the opposite key while accelerating causes a stalling out as the player slows down, until the player ultimately reverses direction. This is going to be called “hard reversing.”
->With some tweaking, “hard reversing” takes less time to do, owing to an increased (and modifiable) acceleration rate during hard reverses. “Hard reversing” still causes skidding and stalling out, which is to be expected.
->Steering implemented
->The player vehicle can move up and down ramps, and gravity affects its movement.
+Laid the foundation for audio for the player character
+Added in a test item, and created basic item detection functionality.
->When the player is close enough, the item begins to glow yellow.
->When the player is facing the item and is close enough, the item glows green, indicating that it can be collected.
->When the player gets out of range of the item, its glow disappears.
+Created a system that enables the player to interact with objects/collect items. It’s dynamic enough to work with anything that requires interacting.
+Implemented item interacting/collecting basic response functionality.
->Whether it is an object or an item the player is interacting with, the basic mechanics are the same: The player goes up to the object/item, faces it, and presses the interact key. From there, something else should happen, whether it is collecting an item, or activating an elevator, etc.
+Created a Level Initializer system, which assigns items to recognize the player and its associated interaction/collection system, so that the player can work with these objects.
-----
Okay, that’s all the additions for this week. So with this first week of development done, I’ve already come across an interesting puzzle that I needed to solve to make things work. For those people who don’t do programming, this might not mean much to you, so just a heads up.
Consider this: In Unity, there are several ways we can make a player object move. We can use Transform.Translate, we can change the rigidbody.velocity to move the character, and we can use rigidbody.MovePosition. Each of these has some interesting quirks, but ask yourself: how do you make a movement system that allows you to the following:
-Make a player go up/down slopes
-Be affected by gravity
-Keeps your player from going through walls
So here’s the thing: Up to this point I had been using rigidbody.velocity changes to do this, and it was working well… but there was one hitch. The gravity of the player object unfortunate enough to get this movement system would barely be affected by gravity - it would slowly… SLOWLY… fall, by decimal places each frame.
Not quite realistic is it?
So this prompted me to investigate a lot of different places. Amidst my search, I discovered something important - that making changes to rigidbody.velocity is basically a big DON’T of Unity programming. Doing so overrides all forces being applied to the rigidbody, including gravity. So, yeah, turns out I have been making that mistake a LOT.
Another thing I’d learned previously was that using transform.translate is not good for physic collision checks either. Yeah, you can do checks manually to ensure it doesn’t go through anything using raycasting and the like, but that’s a lot of extra stuff, and more importantly, using transform.translate is basically causing your object to be teleported. Not moved naturally, even if the movement is just a few decimal places in one direction. As a result, this does not account for collision checks, especially at much higher speeds, when your object will just go past walls, for instance. It’s basically teleporting through them gradually.
I tried using rigidbody.AddForce() as well, but this caused its own set of problems. For one thing, the movement is additive (at least based on what I worked with) - your object keeps getting faster and faster if you don’t set things up right. And if you hit something, there’s a good chance your object (which in this case, was my prototype test player) would end up going into an endless spin. So that ended up not working, either.
So finally, I found something on rigidbody.MovePosition(), which basically moves an object to a new position, while also accounting for collision checks along the way. You can move things in a straight line based on these calculations, and with rigidbody.MoveRotation(), you can rotate your object naturally and account for collision checks as well.
So in summary, rigidbody.MovePosition() and rigidbody.MoveRotation() are a pretty good choice for your 3D player object movement needs. And if you need to teleport in some way, transform.translate will still be of use. But above all else, never, never, NEVER modify rigidbody.velocity. Ever. Not unless you want to mess up your player object’s physics and gravity. It’ll be a source of headaches if you do.
So that’s it from me for now. As a programmer, I’ve seen that the more I learn, the more I realize I still have a lot to learn about programming. It’s not easy to master, and you’ll always have something new to tackle. But hopefully my experience will be of help to someone else who is in my position. If you happen to need information on the various movement options I just explained, going to the Unity Documentation is your best first step.
And this was a very long post, but you can expect these posts to be much shorter on average. In early stages, there is probably more to say on development.